This post is completed by 1 user
|
Add to List |
400. Reverse the Directed Graph
Objective: Given a directed graph, write an algorithm to reverse the graph.
Example:
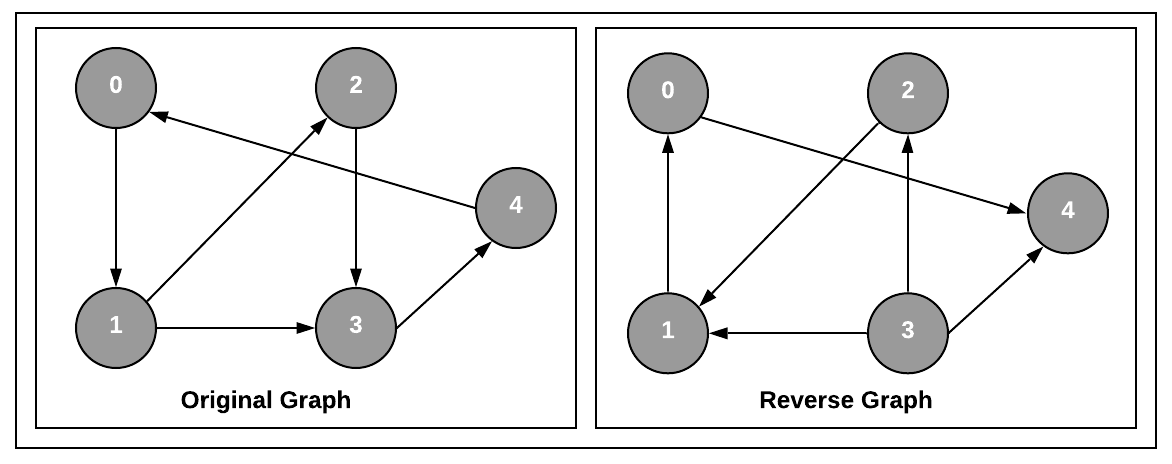
Approach:
Create a new graph with the same number of vertices. Traverse the given graph. Here we are using the adjacency list to represent the graph. Traverse each adjacency list and while traversing keep adding the reverse edges (making source as destination and destination as source). See the code for better understanding.
Output:
---------------Original Graph--------------------------- Vertex connected from vertex: 0 ->1 Vertex connected from vertex: 1 ->3->2 Vertex connected from vertex: 2 ->3 Vertex connected from vertex: 3 ->4 Vertex connected from vertex: 4 ->0 ---------------Reverse Graph--------------------------- Vertex connected from vertex: 0 ->4 Vertex connected from vertex: 1 ->0 Vertex connected from vertex: 2 ->1 Vertex connected from vertex: 3 ->2->1 Vertex connected from vertex: 4 ->3